4 > Produces a string that represents array data in a text table.
6 [](https://travis-ci.org/gajus/table)
7 [](https://coveralls.io/github/gajus/table)
8 [](https://www.npmjs.org/package/table)
9 [](https://github.com/gajus/canonical)
10 [](https://twitter.com/kuizinas)
13 * [Features](#table-features)
14 * [Install](#table-install)
15 * [Usage](#table-usage)
17 * [table](#table-api-table-1)
18 * [createStream](#table-api-createstream)
19 * [getBorderCharacters](#table-api-getbordercharacters)
22 
24 <a name="table-features"></a>
27 * Works with strings containing [fullwidth](https://en.wikipedia.org/wiki/Halfwidth_and_fullwidth_forms) characters.
28 * Works with strings containing [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code).
29 * Configurable border characters.
30 * Configurable content alignment per column.
31 * Configurable content padding per column.
32 * Configurable column width.
35 <a name="table-install"></a>
42 [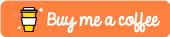](https://www.buymeacoffee.com/gajus)
43 [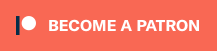](https://www.patreon.com/gajus)
46 <a name="table-usage"></a>
50 import { table } from 'table';
53 // const { table } = require('table');
61 console.log(table(data));
76 <a name="table-api"></a>
79 <a name="table-api-table-1"></a>
82 Returns the string in the table format
85 - **_data_:** The data to display
89 - **_config_:** Table configuration
93 <a name="table-api-table-1-config-border"></a>
96 Type: `{ [type: string]: string }`\
97 Default: `honeywell` [template](#getbordercharacters)
99 Custom borders. The keys are any of:
100 - `topLeft`, `topRight`, `topBody`,`topJoin`
101 - `bottomLeft`, `bottomRight`, `bottomBody`, `bottomJoin`
102 - `joinLeft`, `joinRight`, `joinBody`, `joinJoin`
103 - `bodyLeft`, `bodyRight`, `bodyJoin`
136 console.log(table(data, config));
149 <a name="table-api-table-1-config-drawverticalline"></a>
150 ##### config.drawVerticalLine
152 Type: `(lineIndex: number, columnCount: number) => boolean`\
153 Default: `() => true`
155 It is used to tell whether to draw a vertical line. This callback is called for each vertical border of the table.
156 If the table has `n` columns, then the `index` parameter is alternatively received all numbers in range `[0, n]` inclusively.
168 drawVerticalLine: (lineIndex, columnCount) => {
169 return lineIndex === 0 || lineIndex === columnCount;
173 console.log(table(data, config));
192 <a name="table-api-table-1-config-drawhorizontalline"></a>
193 ##### config.drawHorizontalLine
195 Type: `(lineIndex: number, rowCount: number) => boolean`\
196 Default: `() => true`
198 It is used to tell whether to draw a horizontal line. This callback is called for each horizontal border of the table.
199 If the table has `n` rows, then the `index` parameter is alternatively received all numbers in range `[0, n]` inclusively.
200 If the table has `n` rows and contains the header, then the range will be `[0, n+1]` inclusively.
212 drawHorizontalLine: (lineIndex, rowCount) => {
213 return lineIndex === 0 || lineIndex === 1 || lineIndex === rowCount - 1 || lineIndex === rowCount;
217 console.log(table(data, config));
234 <a name="table-api-table-1-config-singleline"></a>
235 ##### config.singleLine
240 If `true`, horizontal lines inside the table are not drawn. This option also overrides the `config.drawHorizontalLine` if specified.
244 ['-rw-r--r--', '1', 'pandorym', 'staff', '1529', 'May 23 11:25', 'LICENSE'],
245 ['-rw-r--r--', '1', 'pandorym', 'staff', '16327', 'May 23 11:58', 'README.md'],
246 ['drwxr-xr-x', '76', 'pandorym', 'staff', '2432', 'May 23 12:02', 'dist'],
247 ['drwxr-xr-x', '634', 'pandorym', 'staff', '20288', 'May 23 11:54', 'node_modules'],
248 ['-rw-r--r--', '1,', 'pandorym', 'staff', '525688', 'May 23 11:52', 'package-lock.json'],
249 ['-rw-r--r--@', '1', 'pandorym', 'staff', '2440', 'May 23 11:25', 'package.json'],
250 ['drwxr-xr-x', '27', 'pandorym', 'staff', '864', 'May 23 11:25', 'src'],
251 ['drwxr-xr-x', '20', 'pandorym', 'staff', '640', 'May 23 11:25', 'test'],
258 console.log(table(data, config));
262 ╔═════════════╤═════╤══════════╤═══════╤════════╤══════════════╤═══════════════════╗
263 ║ -rw-r--r-- │ 1 │ pandorym │ staff │ 1529 │ May 23 11:25 │ LICENSE ║
264 ║ -rw-r--r-- │ 1 │ pandorym │ staff │ 16327 │ May 23 11:58 │ README.md ║
265 ║ drwxr-xr-x │ 76 │ pandorym │ staff │ 2432 │ May 23 12:02 │ dist ║
266 ║ drwxr-xr-x │ 634 │ pandorym │ staff │ 20288 │ May 23 11:54 │ node_modules ║
267 ║ -rw-r--r-- │ 1, │ pandorym │ staff │ 525688 │ May 23 11:52 │ package-lock.json ║
268 ║ -rw-r--r--@ │ 1 │ pandorym │ staff │ 2440 │ May 23 11:25 │ package.json ║
269 ║ drwxr-xr-x │ 27 │ pandorym │ staff │ 864 │ May 23 11:25 │ src ║
270 ║ drwxr-xr-x │ 20 │ pandorym │ staff │ 640 │ May 23 11:25 │ test ║
271 ╚═════════════╧═════╧══════════╧═══════╧════════╧══════════════╧═══════════════════╝
275 <a name="table-api-table-1-config-columns"></a>
278 Type: `Column[] | { [columnIndex: number]: Column }`
280 Column specific configurations.
282 <a name="table-api-table-1-config-columns-config-columns-width"></a>
283 ###### config.columns[*].width
286 Default: the maximum cell widths of the column
288 Column width (excluding the paddings).
304 console.log(table(data, config));
308 ╔════╤════════════╤════╗
310 ╟────┼────────────┼────╢
312 ╟────┼────────────┼────╢
314 ╚════╧════════════╧════╝
317 <a name="table-api-table-1-config-columns-config-columns-alignment"></a>
318 ###### config.columns[*].alignment
320 Type: `'center' | 'justify' | 'left' | 'right'`\
323 Cell content horizontal alignment
327 ['0A', '0B', '0C', '0D 0E 0F'],
328 ['1A', '1B', '1C', '1D 1E 1F'],
329 ['2A', '2B', '2C', '2D 2E 2F'],
337 { alignment: 'left' },
338 { alignment: 'center' },
339 { alignment: 'right' },
340 { alignment: 'justify' }
344 console.log(table(data, config));
348 ╔════════════╤════════════╤════════════╤════════════╗
349 ║ 0A │ 0B │ 0C │ 0D 0E 0F ║
350 ╟────────────┼────────────┼────────────┼────────────╢
351 ║ 1A │ 1B │ 1C │ 1D 1E 1F ║
352 ╟────────────┼────────────┼────────────┼────────────╢
353 ║ 2A │ 2B │ 2C │ 2D 2E 2F ║
354 ╚════════════╧════════════╧════════════╧════════════╝
357 <a name="table-api-table-1-config-columns-config-columns-verticalalignment"></a>
358 ###### config.columns[*].verticalAlignment
360 Type: `'top' | 'middle' | 'bottom'`\
363 Cell content vertical alignment
367 ['A', 'B', 'C', 'DEF'],
375 { verticalAlignment: 'top' },
376 { verticalAlignment: 'middle' },
377 { verticalAlignment: 'bottom' },
381 console.log(table(data, config));
392 <a name="table-api-table-1-config-columns-config-columns-paddingleft"></a>
393 ###### config.columns[*].paddingLeft
398 The number of whitespaces used to pad the content on the left.
400 <a name="table-api-table-1-config-columns-config-columns-paddingright"></a>
401 ###### config.columns[*].paddingRight
406 The number of whitespaces used to pad the content on the right.
408 The `paddingLeft` and `paddingRight` options do not count on the column width. So the column has `width = 5`, `paddingLeft = 2` and `paddingRight = 2` will have the total width is `9`.
413 ['0A', 'AABBCC', '0C'],
430 console.log(table(data, config));
445 <a name="table-api-table-1-config-columns-config-columns-truncate"></a>
446 ###### config.columns[*].truncate
451 The number of characters is which the content will be truncated.
452 To handle a content that overflows the container width, `table` package implements [text wrapping](#config.columns[*].wrapWord). However, sometimes you may want to truncate content that is too long to be displayed in the table.
456 ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
468 console.log(table(data, config));
472 ╔══════════════════════╗
473 ║ Lorem ipsum dolor si ║
474 ║ t amet, consectetur ║
475 ║ adipiscing elit. Pha ║
476 ║ sellus pulvinar nibh ║
477 ║ sed mauris convall… ║
478 ╚══════════════════════╝
481 <a name="table-api-table-1-config-columns-config-columns-wrapword"></a>
482 ###### config.columns[*].wrapWord
487 The `table` package implements auto text wrapping, i.e., text that has the width greater than the container width will be separated into multiple lines at the nearest space or one of the special characters: `\|/_.,;-`.
489 When `wrapWord` is `false`:
493 ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
497 columns: [ { width: 20 } ]
500 console.log(table(data, config));
504 ╔══════════════════════╗
505 ║ Lorem ipsum dolor si ║
506 ║ t amet, consectetur ║
507 ║ adipiscing elit. Pha ║
508 ║ sellus pulvinar nibh ║
509 ║ sed mauris convallis ║
510 ║ dapibus. Nunc venena ║
511 ║ tis tempus nulla sit ║
513 ╚══════════════════════╝
516 When `wrapWord` is `true`:
519 ╔══════════════════════╗
520 ║ Lorem ipsum dolor ║
524 ║ Phasellus pulvinar ║
526 ║ convallis dapibus. ║
530 ╚══════════════════════╝
535 <a name="table-api-table-1-config-columndefault"></a>
536 ##### config.columnDefault
541 The default configuration for all columns. Column-specific settings will overwrite the default values.
544 <a name="table-api-table-1-config-header"></a>
549 Header configuration.
551 The header configuration inherits the most of the column's, except:
552 - `content` **{string}**: the header content.
553 - `width:` calculate based on the content width automatically.
554 - `alignment:` `center` be default.
555 - `verticalAlignment:` is not supported.
556 - `config.border.topJoin` will be `config.border.topBody` for prettier.
571 content: 'THE HEADER\nThis is the table about something',
575 console.log(table(data, config));
579 ╔══════════════════════════════════════╗
581 ║ This is the table about something ║
582 ╟────────────┬────────────┬────────────╢
584 ╟────────────┼────────────┼────────────╢
586 ╟────────────┼────────────┼────────────╢
588 ╚════════════╧════════════╧════════════╝
592 <a name="table-api-createstream"></a>
595 `table` package exports `createStream` function used to draw a table and append rows.
598 - _**config:**_ the same as `table`'s, except `config.columnDefault.width` and `config.columnCount` must be provided.
602 import { createStream } from 'table';
611 const stream = createStream(config);
614 stream.write([new Date()]);
618 
620 `table` package uses ANSI escape codes to overwrite the output of the last line when a new row is printed.
622 The underlying implementation is explained in this [Stack Overflow answer](http://stackoverflow.com/a/32938658/368691).
624 Streaming supports all of the configuration properties and functionality of a static table (such as auto text wrapping, alignment and padding), e.g.
627 import { createStream } from 'table';
629 import _ from 'lodash';
641 { alignment: 'center' },
647 const stream = createStream(config);
654 random = _.sample('abcdefghijklmnopqrstuvwxyz', _.random(1, 30)).join('');
656 stream.write([i++, new Date(), random]);
660 
663 <a name="table-api-getbordercharacters"></a>
664 ### getBorderCharacters
668 - Type: `'honeywell' | 'norc' | 'ramac' | 'void'`
671 You can load one of the predefined border templates using `getBorderCharacters` function.
674 import { table, getBorderCharacters } from 'table';
683 border: getBorderCharacters(`name of the template`)
686 console.log(table(data, config));
710 # ramac (ASCII; for use in terminals that do not support Unicode characters)
720 # void (no borders; see "borderless table" section of the documentation)
730 Raise [an issue](https://github.com/gajus/table/issues) if you'd like to contribute a new border template.
732 <a name="table-api-getbordercharacters-borderless-table"></a>
733 #### Borderless Table
735 Simply using `void` border character template creates a table with a lot of unnecessary spacing.
737 To create a more pleasant to the eye table, reset the padding and remove the joining rows, e.g.
741 const output = table(data, {
742 border: getBorderCharacters('void'),
747 drawHorizontalLine: () => false