<a name="table"></a>
# Table
+> Produces a string that represents array data in a text table.
+
[](https://travis-ci.org/gajus/table)
[](https://coveralls.io/github/gajus/table)
[](https://www.npmjs.org/package/table)
* [Features](#table-features)
* [Install](#table-install)
* [Usage](#table-usage)
- * [Cell Content Alignment](#table-usage-cell-content-alignment)
- * [Column Width](#table-usage-column-width)
- * [Custom Border](#table-usage-custom-border)
- * [Draw Horizontal Line](#table-usage-draw-horizontal-line)
- * [Single Line Mode](#table-usage-single-line-mode)
- * [Padding Cell Content](#table-usage-padding-cell-content)
- * [Predefined Border Templates](#table-usage-predefined-border-templates)
- * [Streaming](#table-usage-streaming)
- * [Text Truncation](#table-usage-text-truncation)
- * [Text Wrapping](#table-usage-text-wrapping)
-
+ * [API](#table-api)
+ * [table](#table-api-table-1)
+ * [createStream](#table-api-createstream)
+ * [getBorderCharacters](#table-api-getbordercharacters)
-Produces a string that represents array data in a text table.

```bash
npm install table
-
```
[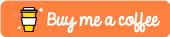](https://www.buymeacoffee.com/gajus)
[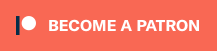](https://www.patreon.com/gajus)
+
<a name="table-usage"></a>
## Usage
-Table data is described using an array (rows) of array (cells).
-
```js
-import {
- table
-} from 'table';
+import { table } from 'table';
// Using commonjs?
-// const {table} = require('table');
-
-let data,
- output;
+// const { table } = require('table');
-data = [
+const data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
-/**
- * @typedef {string} table~cell
- */
-
-/**
- * @typedef {table~cell[]} table~row
- */
-
-/**
- * @typedef {Object} table~columns
- * @property {string} alignment Cell content alignment (enum: left, center, right) (default: left).
- * @property {number} width Column width (default: auto).
- * @property {number} truncate Number of characters are which the content will be truncated (default: Infinity).
- * @property {number} paddingLeft Cell content padding width left (default: 1).
- * @property {number} paddingRight Cell content padding width right (default: 1).
- */
-
-/**
- * @typedef {Object} table~border
- * @property {string} topBody
- * @property {string} topJoin
- * @property {string} topLeft
- * @property {string} topRight
- * @property {string} bottomBody
- * @property {string} bottomJoin
- * @property {string} bottomLeft
- * @property {string} bottomRight
- * @property {string} bodyLeft
- * @property {string} bodyRight
- * @property {string} bodyJoin
- * @property {string} joinBody
- * @property {string} joinLeft
- * @property {string} joinRight
- * @property {string} joinJoin
- */
-
-/**
- * Used to dynamically tell table whether to draw a line separating rows or not.
- * The default behavior is to always return true.
- *
- * @typedef {function} drawHorizontalLine
- * @param {number} index
- * @param {number} size
- * @return {boolean}
- */
-
-/**
- * @typedef {Object} table~config
- * @property {table~border} border
- * @property {table~columns[]} columns Column specific configuration.
- * @property {table~columns} columnDefault Default values for all columns. Column specific settings overwrite the default values.
- * @property {table~drawHorizontalLine} drawHorizontalLine
- */
-
-/**
- * Generates a text table.
- *
- * @param {table~row[]} rows
- * @param {table~config} config
- * @return {String}
- */
-output = table(data);
-
-console.log(output);
+console.log(table(data));
```
```
```
-<a name="table-usage-cell-content-alignment"></a>
-### Cell Content Alignment
+<a name="table-api"></a>
+## API
-`{string} config.columns[{number}].alignment` property controls content horizontal alignment within a cell.
+<a name="table-api-table-1"></a>
+### table
-Valid values are: "left", "right" and "center".
+Returns the string in the table format
-```js
-let config,
- data,
- output;
+**Parameters:**
+- **_data_:** The data to display
+ - Type: `any[][]`
+ - Required: `true`
-data = [
- ['0A', '0B', '0C'],
- ['1A', '1B', '1C'],
- ['2A', '2B', '2C']
-];
-
-config = {
- columns: {
- 0: {
- alignment: 'left',
- width: 10
- },
- 1: {
- alignment: 'center',
- width: 10
- },
- 2: {
- alignment: 'right',
- width: 10
- }
- }
-};
-
-output = table(data, config);
-
-console.log(output);
-```
-
-```
-╔════════════╤════════════╤════════════╗
-║ 0A │ 0B │ 0C ║
-╟────────────┼────────────┼────────────╢
-║ 1A │ 1B │ 1C ║
-╟────────────┼────────────┼────────────╢
-║ 2A │ 2B │ 2C ║
-╚════════════╧════════════╧════════════╝
-```
-
-<a name="table-usage-column-width"></a>
-### Column Width
-
-`{number} config.columns[{number}].width` property restricts column width to a fixed width.
-
-```js
-let data,
- output,
- options;
-
-data = [
- ['0A', '0B', '0C'],
- ['1A', '1B', '1C'],
- ['2A', '2B', '2C']
-];
-
-options = {
- columns: {
- 1: {
- width: 10
- }
- }
-};
-
-output = table(data, options);
-
-console.log(output);
-```
+- **_config_:** Table configuration
+ - Type: `object`
+ - Required: `false`
-```
-╔════╤════════════╤════╗
-║ 0A │ 0B │ 0C ║
-╟────┼────────────┼────╢
-║ 1A │ 1B │ 1C ║
-╟────┼────────────┼────╢
-║ 2A │ 2B │ 2C ║
-╚════╧════════════╧════╝
-```
+<a name="table-api-table-1-config-border"></a>
+##### config.border
-<a name="table-usage-custom-border"></a>
-### Custom Border
+Type: `{ [type: string]: string }`\
+Default: `honeywell` [template](#getbordercharacters)
-`{object} config.border` property describes characters used to draw the table border.
+Custom borders. The keys are any of:
+- `topLeft`, `topRight`, `topBody`,`topJoin`
+- `bottomLeft`, `bottomRight`, `bottomBody`, `bottomJoin`
+- `joinLeft`, `joinRight`, `joinBody`, `joinJoin`
+- `bodyLeft`, `bodyRight`, `bodyJoin`
+- `headerJoin`
```js
-let config,
- data,
- output;
-
-data = [
+const data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
-config = {
+const config = {
border: {
topBody: `─`,
topJoin: `┬`,
}
};
-output = table(data, config);
-
-console.log(output);
+console.log(table(data, config));
```
```
└────┴────┴────┘
```
-<a name="table-usage-draw-horizontal-line"></a>
-### Draw Horizontal Line
+<a name="table-api-table-1-config-drawverticalline"></a>
+##### config.drawVerticalLine
-`{function} config.drawHorizontalLine` property is a function that is called for every non-content row in the table. The result of the function `{boolean}` determines whether a row is drawn.
+Type: `(lineIndex: number, columnCount: number) => boolean`\
+Default: `() => true`
-```js
-let data,
- output,
- options;
+It is used to tell whether to draw a vertical line. This callback is called for each vertical border of the table.
+If the table has `n` columns, then the `index` parameter is alternatively received all numbers in range `[0, n]` inclusively.
-data = [
+```js
+const data = [
['0A', '0B', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C'],
['4A', '4B', '4C']
];
-options = {
- /**
- * @typedef {function} drawHorizontalLine
- * @param {number} index
- * @param {number} size
- * @return {boolean}
- */
- drawHorizontalLine: (index, size) => {
- return index === 0 || index === 1 || index === size - 1 || index === size;
+const config = {
+ drawVerticalLine: (lineIndex, columnCount) => {
+ return lineIndex === 0 || lineIndex === columnCount;
}
};
-output = table(data, options);
+console.log(table(data, config));
-console.log(output);
+```
+
+```
+╔════════════╗
+║ 0A 0B 0C ║
+╟────────────╢
+║ 1A 1B 1C ║
+╟────────────╢
+║ 2A 2B 2C ║
+╟────────────╢
+║ 3A 3B 3C ║
+╟────────────╢
+║ 4A 4B 4C ║
+╚════════════╝
+
+```
+
+<a name="table-api-table-1-config-drawhorizontalline"></a>
+##### config.drawHorizontalLine
+
+Type: `(lineIndex: number, rowCount: number) => boolean`\
+Default: `() => true`
+
+It is used to tell whether to draw a horizontal line. This callback is called for each horizontal border of the table.
+If the table has `n` rows, then the `index` parameter is alternatively received all numbers in range `[0, n]` inclusively.
+If the table has `n` rows and contains the header, then the range will be `[0, n+1]` inclusively.
+
+```js
+const data = [
+ ['0A', '0B', '0C'],
+ ['1A', '1B', '1C'],
+ ['2A', '2B', '2C'],
+ ['3A', '3B', '3C'],
+ ['4A', '4B', '4C']
+];
+
+const config = {
+ drawHorizontalLine: (lineIndex, rowCount) => {
+ return lineIndex === 0 || lineIndex === 1 || lineIndex === rowCount - 1 || lineIndex === rowCount;
+ }
+};
+
+console.log(table(data, config));
```
```
-<a name="table-usage-single-line-mode"></a>
-### Single Line Mode
+<a name="table-api-table-1-config-singleline"></a>
+##### config.singleLine
-Horizontal lines inside the table are not drawn.
+Type: `boolean`\
+Default: `false`
-```js
-import {
- table,
- getBorderCharacters
-} from 'table';
+If `true`, horizontal lines inside the table are not drawn. This option also overrides the `config.drawHorizontalLine` if specified.
+```js
const data = [
['-rw-r--r--', '1', 'pandorym', 'staff', '1529', 'May 23 11:25', 'LICENSE'],
['-rw-r--r--', '1', 'pandorym', 'staff', '16327', 'May 23 11:58', 'README.md'],
singleLine: true
};
-const output = table(data, config);
-console.log(output);
+console.log(table(data, config));
```
```
╚═════════════╧═════╧══════════╧═══════╧════════╧══════════════╧═══════════════════╝
```
-<a name="table-usage-padding-cell-content"></a>
-### Padding Cell Content
-`{number} config.columns[{number}].paddingLeft` and `{number} config.columns[{number}].paddingRight` properties control content padding within a cell. Property value represents a number of whitespaces used to pad the content.
+<a name="table-api-table-1-config-columns"></a>
+##### config.columns
+
+Type: `Column[] | { [columnIndex: number]: Column }`
+
+Column specific configurations.
+
+<a name="table-api-table-1-config-columns-config-columns-width"></a>
+###### config.columns[*].width
+
+Type: `number`\
+Default: the maximum cell widths of the column
+
+Column width (excluding the paddings).
+
+```js
+
+const data = [
+ ['0A', '0B', '0C'],
+ ['1A', '1B', '1C'],
+ ['2A', '2B', '2C']
+];
+
+const config = {
+ columns: {
+ 1: { width: 10 }
+ }
+};
+
+console.log(table(data, config));
+```
+
+```
+╔════╤════════════╤════╗
+║ 0A │ 0B │ 0C ║
+╟────┼────────────┼────╢
+║ 1A │ 1B │ 1C ║
+╟────┼────────────┼────╢
+║ 2A │ 2B │ 2C ║
+╚════╧════════════╧════╝
+```
+
+<a name="table-api-table-1-config-columns-config-columns-alignment"></a>
+###### config.columns[*].alignment
+
+Type: `'center' | 'justify' | 'left' | 'right'`\
+Default: `'left'`
+
+Cell content horizontal alignment
+
+```js
+const data = [
+ ['0A', '0B', '0C', '0D 0E 0F'],
+ ['1A', '1B', '1C', '1D 1E 1F'],
+ ['2A', '2B', '2C', '2D 2E 2F'],
+];
+
+const config = {
+ columnDefault: {
+ width: 10,
+ },
+ columns: [
+ { alignment: 'left' },
+ { alignment: 'center' },
+ { alignment: 'right' },
+ { alignment: 'justify' }
+ ],
+};
+
+console.log(table(data, config));
+```
+
+```
+╔════════════╤════════════╤════════════╤════════════╗
+║ 0A │ 0B │ 0C │ 0D 0E 0F ║
+╟────────────┼────────────┼────────────┼────────────╢
+║ 1A │ 1B │ 1C │ 1D 1E 1F ║
+╟────────────┼────────────┼────────────┼────────────╢
+║ 2A │ 2B │ 2C │ 2D 2E 2F ║
+╚════════════╧════════════╧════════════╧════════════╝
+```
+
+<a name="table-api-table-1-config-columns-config-columns-verticalalignment"></a>
+###### config.columns[*].verticalAlignment
+
+Type: `'top' | 'middle' | 'bottom'`\
+Default: `'top'`
+
+Cell content vertical alignment
```js
-let config,
- data,
- output;
+const data = [
+ ['A', 'B', 'C', 'DEF'],
+];
+
+const config = {
+ columnDefault: {
+ width: 1,
+ },
+ columns: [
+ { verticalAlignment: 'top' },
+ { verticalAlignment: 'middle' },
+ { verticalAlignment: 'bottom' },
+ ],
+};
+
+console.log(table(data, config));
+```
+
+```
+╔═══╤═══╤═══╤═══╗
+║ A │ │ │ D ║
+║ │ B │ │ E ║
+║ │ │ C │ F ║
+╚═══╧═══╧═══╧═══╝
+```
+
+<a name="table-api-table-1-config-columns-config-columns-paddingleft"></a>
+###### config.columns[*].paddingLeft
+
+Type: `number`\
+Default: `1`
+
+The number of whitespaces used to pad the content on the left.
+
+<a name="table-api-table-1-config-columns-config-columns-paddingright"></a>
+###### config.columns[*].paddingRight
+
+Type: `number`\
+Default: `1`
-data = [
+The number of whitespaces used to pad the content on the right.
+
+The `paddingLeft` and `paddingRight` options do not count on the column width. So the column has `width = 5`, `paddingLeft = 2` and `paddingRight = 2` will have the total width is `9`.
+
+
+```js
+const data = [
['0A', 'AABBCC', '0C'],
['1A', '1B', '1C'],
['2A', '2B', '2C']
];
-config = {
- columns: {
- 0: {
+const config = {
+ columns: [
+ {
paddingLeft: 3
},
- 1: {
+ {
width: 2,
paddingRight: 3
}
- }
+ ]
};
-output = table(data, config);
-
-console.log(output);
+console.log(table(data, config));
```
```
╚══════╧══════╧════╝
```
-<a name="table-usage-predefined-border-templates"></a>
-### Predefined Border Templates
+<a name="table-api-table-1-config-columns-config-columns-truncate"></a>
+###### config.columns[*].truncate
-You can load one of the predefined border templates using `getBorderCharacters` function.
+Type: `number`\
+Default: `Infinity`
-```js
-import {
- table,
- getBorderCharacters
-} from 'table';
+The number of characters is which the content will be truncated.
+To handle a content that overflows the container width, `table` package implements [text wrapping](#config.columns[*].wrapWord). However, sometimes you may want to truncate content that is too long to be displayed in the table.
-let config,
- data;
-
-data = [
- ['0A', '0B', '0C'],
- ['1A', '1B', '1C'],
- ['2A', '2B', '2C']
+```js
+const data = [
+ ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
];
-config = {
- border: getBorderCharacters(`name of the template`)
+const config = {
+ columns: [
+ {
+ width: 20,
+ truncate: 100
+ }
+ ]
};
-table(data, config);
+console.log(table(data, config));
```
```
-# honeywell
+╔══════════════════════╗
+║ Lorem ipsum dolor si ║
+║ t amet, consectetur ║
+║ adipiscing elit. Pha ║
+║ sellus pulvinar nibh ║
+║ sed mauris convall… ║
+╚══════════════════════╝
+```
-╔════╤════╤════╗
-║ 0A │ 0B │ 0C ║
-╟────┼────┼────╢
-║ 1A │ 1B │ 1C ║
-╟────┼────┼────╢
-║ 2A │ 2B │ 2C ║
-╚════╧════╧════╝
+<a name="table-api-table-1-config-columns-config-columns-wrapword"></a>
+###### config.columns[*].wrapWord
-# norc
+Type: `boolean`\
+Default: `false`
-┌────┬────┬────┐
-│ 0A │ 0B │ 0C │
-├────┼────┼────┤
-│ 1A │ 1B │ 1C │
-├────┼────┼────┤
-│ 2A │ 2B │ 2C │
-└────┴────┴────┘
+The `table` package implements auto text wrapping, i.e., text that has the width greater than the container width will be separated into multiple lines at the nearest space or one of the special characters: `\|/_.,;-`.
-# ramac (ASCII; for use in terminals that do not support Unicode characters)
+When `wrapWord` is `false`:
-+----+----+----+
-| 0A | 0B | 0C |
-|----|----|----|
-| 1A | 1B | 1C |
-|----|----|----|
-| 2A | 2B | 2C |
-+----+----+----+
+```js
+const data = [
+ ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
+];
-# void (no borders; see "bordless table" section of the documentation)
+const config = {
+ columns: [ { width: 20 } ]
+};
- 0A 0B 0C
+console.log(table(data, config));
+```
- 1A 1B 1C
+```
+╔══════════════════════╗
+║ Lorem ipsum dolor si ║
+║ t amet, consectetur ║
+║ adipiscing elit. Pha ║
+║ sellus pulvinar nibh ║
+║ sed mauris convallis ║
+║ dapibus. Nunc venena ║
+║ tis tempus nulla sit ║
+║ amet viverra. ║
+╚══════════════════════╝
+```
- 2A 2B 2C
+When `wrapWord` is `true`:
+
+```
+╔══════════════════════╗
+║ Lorem ipsum dolor ║
+║ sit amet, ║
+║ consectetur ║
+║ adipiscing elit. ║
+║ Phasellus pulvinar ║
+║ nibh sed mauris ║
+║ convallis dapibus. ║
+║ Nunc venenatis ║
+║ tempus nulla sit ║
+║ amet viverra. ║
+╚══════════════════════╝
```
-Raise [an issue](https://github.com/gajus/table/issues) if you'd like to contribute a new border template.
-<a name="table-usage-predefined-border-templates-borderless-table"></a>
-#### Borderless Table
+<a name="table-api-table-1-config-columndefault"></a>
+##### config.columnDefault
+
+Type: `Column`\
+Default: `{}`
+
+The default configuration for all columns. Column-specific settings will overwrite the default values.
+
+
+<a name="table-api-table-1-config-header"></a>
+##### config.header
+
+Type: `object`
-Simply using "void" border character template creates a table with a lot of unnecessary spacing.
+Header configuration.
-To create a more plesant to the eye table, reset the padding and remove the joining rows, e.g.
+The header configuration inherits the most of the column's, except:
+- `content` **{string}**: the header content.
+- `width:` calculate based on the content width automatically.
+- `alignment:` `center` be default.
+- `verticalAlignment:` is not supported.
+- `config.border.topJoin` will be `config.border.topBody` for prettier.
```js
-let output;
+const data = [
+ ['0A', '0B', '0C'],
+ ['1A', '1B', '1C'],
+ ['2A', '2B', '2C'],
+ ];
-output = table(data, {
- border: getBorderCharacters(`void`),
- columnDefault: {
- paddingLeft: 0,
- paddingRight: 1
- },
- drawHorizontalLine: () => {
- return false
- }
-});
+const config = {
+ columnDefault: {
+ width: 10,
+ },
+ header: {
+ alignment: 'center',
+ content: 'THE HEADER\nThis is the table about something',
+ },
+}
-console.log(output);
+console.log(table(data, config));
```
```
-0A 0B 0C
-1A 1B 1C
-2A 2B 2C
+╔══════════════════════════════════════╗
+║ THE HEADER ║
+║ This is the table about something ║
+╟────────────┬────────────┬────────────╢
+║ 0A │ 0B │ 0C ║
+╟────────────┼────────────┼────────────╢
+║ 1A │ 1B │ 1C ║
+╟────────────┼────────────┼────────────╢
+║ 2A │ 2B │ 2C ║
+╚════════════╧════════════╧════════════╝
```
-<a name="table-usage-streaming"></a>
-### Streaming
+
+<a name="table-api-createstream"></a>
+### createStream
`table` package exports `createStream` function used to draw a table and append rows.
-`createStream` requires `{number} columnDefault.width` and `{number} columnCount` configuration properties.
+**Parameter:**
+ - _**config:**_ the same as `table`'s, except `config.columnDefault.width` and `config.columnCount` must be provided.
-```js
-import {
- createStream
-} from 'table';
-let config,
- stream;
+```js
+import { createStream } from 'table';
-config = {
+const config = {
columnDefault: {
width: 50
},
columnCount: 1
};
-stream = createStream(config);
+const stream = createStream(config);
setInterval(() => {
stream.write([new Date()]);
}, 500);
```
-
+
`table` package uses ANSI escape codes to overwrite the output of the last line when a new row is printed.
Streaming supports all of the configuration properties and functionality of a static table (such as auto text wrapping, alignment and padding), e.g.
```js
-import {
- createStream
-} from 'table';
+import { createStream } from 'table';
import _ from 'lodash';
-let config,
- stream,
- i;
-
-config = {
+const config = {
columnDefault: {
width: 50
},
columnCount: 3,
- columns: {
- 0: {
+ columns: [
+ {
width: 10,
alignment: 'right'
},
- 1: {
- alignment: 'center',
- },
- 2: {
- width: 10
- }
- }
+ { alignment: 'center' },
+ { width: 10 }
+
+ ]
};
-stream = createStream(config);
+const stream = createStream(config);
-i = 0;
+let i = 0;
setInterval(() => {
let random;
}, 500);
```
-
+
+
-<a name="table-usage-text-truncation"></a>
-### Text Truncation
+<a name="table-api-getbordercharacters"></a>
+### getBorderCharacters
-To handle a content that overflows the container width, `table` package implements [text wrapping](#table-usage-text-wrapping). However, sometimes you may want to truncate content that is too long to be displayed in the table.
+**Parameter:**
+ - **_template_**
+ - Type: `'honeywell' | 'norc' | 'ramac' | 'void'`
+ - Required: `true`
-`{number} config.columns[{number}].truncate` property (default: `Infinity`) truncates the text at the specified length.
+You can load one of the predefined border templates using `getBorderCharacters` function.
```js
-let config,
- data,
- output;
+import { table, getBorderCharacters } from 'table';
-data = [
- ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
+const data = [
+ ['0A', '0B', '0C'],
+ ['1A', '1B', '1C'],
+ ['2A', '2B', '2C']
];
-config = {
- columns: {
- 0: {
- width: 20,
- truncate: 100
- }
- }
+const config = {
+ border: getBorderCharacters(`name of the template`)
};
-output = table(data, config);
-
-console.log(output);
+console.log(table(data, config));
```
```
-╔══════════════════════╗
-║ Lorem ipsum dolor si ║
-║ t amet, consectetur ║
-║ adipiscing elit. Pha ║
-║ sellus pulvinar nibh ║
-║ sed mauris conva... ║
-╚══════════════════════╝
-```
+# honeywell
-<a name="table-usage-text-wrapping"></a>
-### Text Wrapping
+╔════╤════╤════╗
+║ 0A │ 0B │ 0C ║
+╟────┼────┼────╢
+║ 1A │ 1B │ 1C ║
+╟────┼────┼────╢
+║ 2A │ 2B │ 2C ║
+╚════╧════╧════╝
-`table` package implements auto text wrapping, i.e. text that has width greater than the container width will be separated into multiple lines, e.g.
+# norc
-```js
-let config,
- data,
- output;
+┌────┬────┬────┐
+│ 0A │ 0B │ 0C │
+├────┼────┼────┤
+│ 1A │ 1B │ 1C │
+├────┼────┼────┤
+│ 2A │ 2B │ 2C │
+└────┴────┴────┘
-data = [
- ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
-];
+# ramac (ASCII; for use in terminals that do not support Unicode characters)
-config = {
- columns: {
- 0: {
- width: 20
- }
- }
-};
++----+----+----+
+| 0A | 0B | 0C |
+|----|----|----|
+| 1A | 1B | 1C |
+|----|----|----|
+| 2A | 2B | 2C |
++----+----+----+
-output = table(data, config);
+# void (no borders; see "borderless table" section of the documentation)
-console.log(output);
-```
+ 0A 0B 0C
+
+ 1A 1B 1C
+
+ 2A 2B 2C
-```
-╔══════════════════════╗
-║ Lorem ipsum dolor si ║
-║ t amet, consectetur ║
-║ adipiscing elit. Pha ║
-║ sellus pulvinar nibh ║
-║ sed mauris convallis ║
-║ dapibus. Nunc venena ║
-║ tis tempus nulla sit ║
-║ amet viverra. ║
-╚══════════════════════╝
```
-When `wrapWord` is `true` the text is broken at the nearest space or one of the special characters ("-", "_", "\", "/", ".", ",", ";"), e.g.
+Raise [an issue](https://github.com/gajus/table/issues) if you'd like to contribute a new border template.
-```js
-let config,
- data,
- output;
+<a name="table-api-getbordercharacters-borderless-table"></a>
+#### Borderless Table
-data = [
- ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
-];
+Simply using `void` border character template creates a table with a lot of unnecessary spacing.
-config = {
- columns: {
- 0: {
- width: 20,
- wrapWord: true
- }
- }
-};
+To create a more pleasant to the eye table, reset the padding and remove the joining rows, e.g.
+
+```js
-output = table(data, config);
+const output = table(data, {
+ border: getBorderCharacters('void'),
+ columnDefault: {
+ paddingLeft: 0,
+ paddingRight: 1
+ },
+ drawHorizontalLine: () => false
+ }
+);
console.log(output);
```
```
-╔══════════════════════╗
-║ Lorem ipsum dolor ║
-║ sit amet, ║
-║ consectetur ║
-║ adipiscing elit. ║
-║ Phasellus pulvinar ║
-║ nibh sed mauris ║
-║ convallis dapibus. ║
-║ Nunc venenatis ║
-║ tempus nulla sit ║
-║ amet viverra. ║
-╚══════════════════════╝
-
+0A 0B 0C
+1A 1B 1C
+2A 2B 2C
```