2 [](https://travis-ci.org/Marak/colors.js)
3 [](https://www.npmjs.org/package/colors)
4 [](https://david-dm.org/Marak/colors.js)
5 [](https://david-dm.org/Marak/colors.js#info=devDependencies)
7 Please check out the [roadmap](ROADMAP.md) for upcoming features and releases. Please open Issues to provide feedback, and check the `develop` branch for the latest bleeding-edge updates.
9 ## get color and style in your node.js console
11 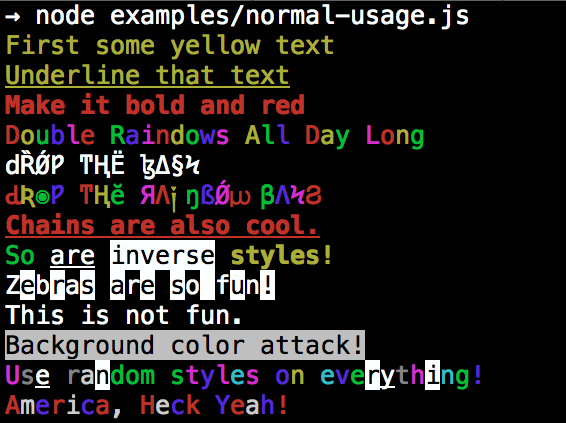
32 ### bright text colors
55 ### bright background colors
87 By popular demand, `colors` now ships with two types of usages!
92 var colors = require('colors');
94 console.log('hello'.green); // outputs green text
95 console.log('i like cake and pies'.underline.red) // outputs red underlined text
96 console.log('inverse the color'.inverse); // inverses the color
97 console.log('OMG Rainbows!'.rainbow); // rainbow
98 console.log('Run the trap'.trap); // Drops the bass
102 or a slightly less nifty way which doesn't extend `String.prototype`
105 var colors = require('colors/safe');
107 console.log(colors.green('hello')); // outputs green text
108 console.log(colors.red.underline('i like cake and pies')) // outputs red underlined text
109 console.log(colors.inverse('inverse the color')); // inverses the color
110 console.log(colors.rainbow('OMG Rainbows!')); // rainbow
111 console.log(colors.trap('Run the trap')); // Drops the bass
115 I prefer the first way. Some people seem to be afraid of extending `String.prototype` and prefer the second way.
117 If you are writing good code you will never have an issue with the first approach. If you really don't want to touch `String.prototype`, the second usage will not touch `String` native object.
119 ## Enabling/Disabling Colors
121 The package will auto-detect whether your terminal can use colors and enable/disable accordingly. When colors are disabled, the color functions do nothing. You can override this with a command-line flag:
124 node myapp.js --no-color
125 node myapp.js --color=false
127 node myapp.js --color
128 node myapp.js --color=true
129 node myapp.js --color=always
131 FORCE_COLOR=1 node myapp.js
137 var colors = require('colors');
142 ## Console.log [string substitution](http://nodejs.org/docs/latest/api/console.html#console_console_log_data)
146 console.log(colors.green('Hello %s'), name);
147 // outputs -> 'Hello Marak'
152 ### Using standard API
156 var colors = require('colors');
172 console.log("this is an error".error);
174 // outputs yellow text
175 console.log("this is a warning".warn);
178 ### Using string safe API
181 var colors = require('colors/safe');
183 // set single property
184 var error = colors.red;
185 error('this is red');
202 console.log(colors.error("this is an error"));
204 // outputs yellow text
205 console.log(colors.warn("this is a warning"));
212 var colors = require('colors');
215 custom: ['red', 'underline']
218 console.log('test'.custom);
221 *Protip: There is a secret undocumented style in `colors`. If you find the style you can summon him.*