1 # ansi-colors [](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=W8YFZ425KND68) [](https://www.npmjs.com/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://npmjs.org/package/ansi-colors) [](https://travis-ci.org/doowb/ansi-colors)
3 > Easily add ANSI colors to your text and symbols in the terminal. A faster drop-in replacement for chalk, kleur and turbocolor (without the dependencies and rendering bugs).
5 Please consider following this project's author, [Brian Woodward](https://github.com/doowb), and consider starring the project to show your :heart: and support.
9 Install with [npm](https://www.npmjs.com/):
12 $ npm install --save ansi-colors
15 
19 ansi-colors is _the fastest Node.js library for terminal styling_. A more performant drop-in replacement for chalk, with no dependencies.
21 * _Blazing fast_ - Fastest terminal styling library in node.js, 10-20x faster than chalk!
23 * _Drop-in replacement_ for [chalk](https://github.com/chalk/chalk).
24 * _No dependencies_ (Chalk has 7 dependencies in its tree!)
26 * _Safe_ - Does not modify the `String.prototype` like [colors](https://github.com/Marak/colors.js).
27 * Supports [nested colors](#nested-colors), **and does not have the [nested styling bug](#nested-styling-bug) that is present in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur)**.
28 * Supports [chained colors](#chained-colors).
29 * [Toggle color support](#toggle-color-support) on or off.
34 const c = require('ansi-colors');
36 console.log(c.red('This is a red string!'));
37 console.log(c.green('This is a red string!'));
38 console.log(c.cyan('This is a cyan string!'));
39 console.log(c.yellow('This is a yellow string!'));
42 
47 console.log(c.bold.red('this is a bold red message'));
48 console.log(c.bold.yellow.italic('this is a bold yellow italicized message'));
49 console.log(c.green.bold.underline('this is a bold green underlined message'));
52 
57 console.log(c.yellow(`foo ${c.red.bold('red')} bar ${c.cyan('cyan')} baz`));
60 
62 ### Nested styling bug
64 `ansi-colors` does not have the nested styling bug found in [colorette](https://github.com/jorgebucaran/colorette), [chalk](https://github.com/chalk/chalk), and [kleur](https://github.com/lukeed/kleur).
67 const { bold, red } = require('ansi-styles');
68 console.log(bold(`foo ${red.dim('bar')} baz`));
70 const colorette = require('colorette');
71 console.log(colorette.bold(`foo ${colorette.red(colorette.dim('bar'))} baz`));
73 const kleur = require('kleur');
74 console.log(kleur.bold(`foo ${kleur.red.dim('bar')} baz`));
76 const chalk = require('chalk');
77 console.log(chalk.bold(`foo ${chalk.red.dim('bar')} baz`));
80 **Results in the following**
82 (sans icons and labels)
84 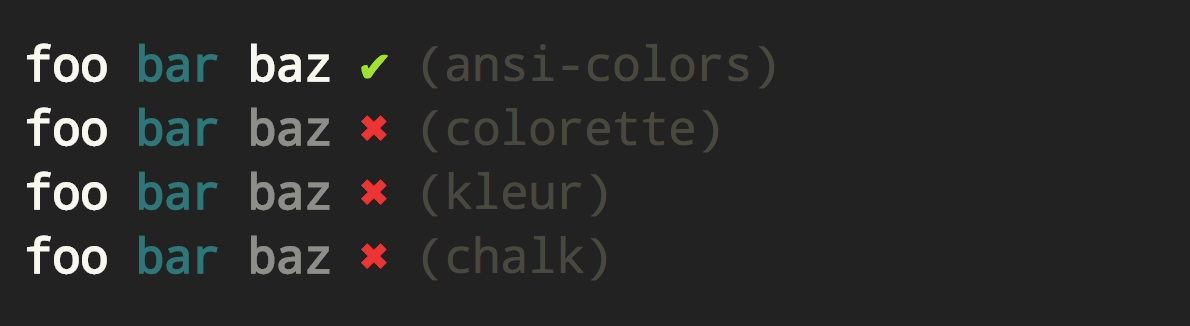
86 ## Toggle color support
88 Easily enable/disable colors.
91 const c = require('ansi-colors');
93 // disable colors manually
96 // or use a library to automatically detect support
97 c.enabled = require('color-support').hasBasic;
99 console.log(c.red('I will only be colored red if the terminal supports colors'));
104 Use the `.unstyle` method to strip ANSI codes from a string.
107 console.log(c.unstyle(c.blue.bold('foo bar baz')));
113 **Note** that bright and bright-background colors are not always supported.
115 | Colors | Background Colors | Bright Colors | Bright Background Colors |
116 | ------- | ----------------- | ------------- | ------------------------ |
117 | black | bgBlack | blackBright | bgBlackBright |
118 | red | bgRed | redBright | bgRedBright |
119 | green | bgGreen | greenBright | bgGreenBright |
120 | yellow | bgYellow | yellowBright | bgYellowBright |
121 | blue | bgBlue | blueBright | bgBlueBright |
122 | magenta | bgMagenta | magentaBright | bgMagentaBright |
123 | cyan | bgCyan | cyanBright | bgCyanBright |
124 | white | bgWhite | whiteBright | bgWhiteBright |
128 _(`gray` is the U.S. spelling, `grey` is more commonly used in the Canada and U.K.)_
146 Create custom aliases for styles.
149 const colors = require('ansi-colors');
151 colors.alias('primary', colors.yellow);
152 colors.alias('secondary', colors.bold);
154 console.log(colors.primary.secondary('Foo'));
159 A theme is an object of custom aliases.
162 const colors = require('ansi-colors');
166 dark: colors.dim.gray,
167 disabled: colors.gray,
169 heading: colors.bold.underline,
172 primary: colors.blue,
174 success: colors.green,
175 underline: colors.underline,
176 warning: colors.yellow
179 // Now, we can use our custom styles alongside the built-in styles!
180 console.log(colors.danger.strong.em('Error!'));
181 console.log(colors.warning('Heads up!'));
182 console.log(colors.info('Did you know...'));
183 console.log(colors.success.bold('It worked!'));
195 > MacBook Pro, Intel Core i7, 2.3 GHz, 16 GB.
199 Time it takes to load the first time `require()` is called:
201 * ansi-colors - `1.915ms`
208 ansi-colors x 173,851 ops/sec ±0.42% (91 runs sampled)
209 chalk x 9,944 ops/sec ±2.53% (81 runs sampled)))
212 ansi-colors x 20,791 ops/sec ±0.60% (88 runs sampled)
213 chalk x 2,111 ops/sec ±2.34% (83 runs sampled)
216 ansi-colors x 59,304 ops/sec ±0.98% (92 runs sampled)
217 chalk x 4,590 ops/sec ±2.08% (82 runs sampled)
222 > Windows 10, Intel Core i7-7700k CPU @ 4.2 GHz, 32 GB
226 Time it takes to load the first time `require()` is called:
228 * ansi-colors - `1.494ms`
235 ansi-colors x 193,088 ops/sec ±0.51% (95 runs sampled))
236 chalk x 9,612 ops/sec ±3.31% (77 runs sampled)))
239 ansi-colors x 26,093 ops/sec ±1.13% (94 runs sampled)
240 chalk x 2,267 ops/sec ±2.88% (80 runs sampled))
243 ansi-colors x 67,747 ops/sec ±0.49% (93 runs sampled)
244 chalk x 4,446 ops/sec ±3.01% (82 runs sampled))
250 <summary><strong>Contributing</strong></summary>
252 Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
257 <summary><strong>Running Tests</strong></summary>
259 Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
262 $ npm install && npm test
268 <summary><strong>Building docs</strong></summary>
270 _(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
272 To generate the readme, run the following command:
275 $ npm install -g verbose/verb#dev verb-generate-readme && verb
282 You might also be interested in these projects:
284 * [ansi-wrap](https://www.npmjs.com/package/ansi-wrap): Create ansi colors by passing the open and close codes. | [homepage](https://github.com/jonschlinkert/ansi-wrap "Create ansi colors by passing the open and close codes.")
285 * [strip-color](https://www.npmjs.com/package/strip-color): Strip ANSI color codes from a string. No dependencies. | [homepage](https://github.com/jonschlinkert/strip-color "Strip ANSI color codes from a string. No dependencies.")
289 | **Commits** | **Contributor** |
291 | 48 | [jonschlinkert](https://github.com/jonschlinkert) |
292 | 42 | [doowb](https://github.com/doowb) |
293 | 6 | [lukeed](https://github.com/lukeed) |
294 | 2 | [Silic0nS0ldier](https://github.com/Silic0nS0ldier) |
295 | 1 | [dwieeb](https://github.com/dwieeb) |
296 | 1 | [jorgebucaran](https://github.com/jorgebucaran) |
297 | 1 | [madhavarshney](https://github.com/madhavarshney) |
298 | 1 | [chapterjason](https://github.com/chapterjason) |
304 * [GitHub Profile](https://github.com/doowb)
305 * [Twitter Profile](https://twitter.com/doowb)
306 * [LinkedIn Profile](https://linkedin.com/in/woodwardbrian)
310 Copyright © 2019, [Brian Woodward](https://github.com/doowb).
311 Released under the [MIT License](LICENSE).
315 _This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.8.0, on July 01, 2019._