1 glob-parent [](https://travis-ci.org/es128/glob-parent) [](https://coveralls.io/r/es128/glob-parent?branch=master)
3 Javascript module to extract the non-magic parent path from a glob string.
5 [](https://nodei.co/npm/glob-parent/)
6 [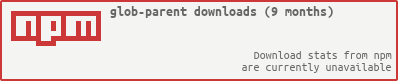](https://nodei.co/npm-dl/glob-parent/)
11 npm install glob-parent --save
17 var globParent = require('glob-parent');
19 globParent('path/to/*.js'); // 'path/to'
20 globParent('/root/path/to/*.js'); // '/root/path/to'
21 globParent('/*.js'); // '/'
22 globParent('*.js'); // '.'
23 globParent('**/*.js'); // '.'
24 globParent('path/{to,from}'); // 'path'
25 globParent('path/!(to|from)'); // 'path'
26 globParent('path/?(to|from)'); // 'path'
27 globParent('path/+(to|from)'); // 'path'
28 globParent('path/*(to|from)'); // 'path'
29 globParent('path/@(to|from)'); // 'path'
30 globParent('path/**/*'); // 'path'
32 // if provided a non-glob path, returns the nearest dir
33 globParent('path/foo/bar.js'); // 'path/foo'
34 globParent('path/foo/'); // 'path/foo'
35 globParent('path/foo'); // 'path' (see issue #3 for details)
40 The following characters have special significance in glob patterns and must be escaped if you want them to be treated as regular path characters:
45 - `(` (opening parenthesis)
46 - `)` (closing parenthesis)
47 - `{` (opening curly brace)
48 - `}` (closing curly brace)
49 - `[` (opening bracket)
50 - `]` (closing bracket)
55 globParent('foo/[bar]/') // 'foo'
56 globParent('foo/\\[bar]/') // 'foo/[bar]'
61 #### Braces & Brackets
62 This library attempts a quick and imperfect method of determining which path
63 parts have glob magic without fully parsing/lexing the pattern. There are some
64 advanced use cases that can trip it up, such as nested braces where the outer
65 pair is escaped and the inner one contains a path separator. If you find
66 yourself in the unlikely circumstance of being affected by this or need to
67 ensure higher-fidelity glob handling in your library, it is recommended that you
68 pre-process your input with [expand-braces] and/or [expand-brackets].
71 Backslashes are not valid path separators for globs. If a path with backslashes
72 is provided anyway, for simple cases, glob-parent will replace the path
73 separator for you and return the non-glob parent path (now with
74 forward-slashes, which are still valid as Windows path separators).
76 This cannot be used in conjunction with escape characters.
80 globParent('C:\\Program Files \\(x86\\)\\*.ext') // 'C:/Program Files /(x86/)'
83 globParent('C:/Program Files\\(x86\\)/*.ext') // 'C:/Program Files (x86)'
86 If you are using escape characters for a pattern without path parts (i.e.
87 relative to `cwd`), prefix with `./` to avoid confusing glob-parent.
91 globParent('foo \\[bar]') // 'foo '
92 globParent('foo \\[bar]*') // 'foo '
95 globParent('./foo \\[bar]') // 'foo [bar]'
96 globParent('./foo \\[bar]*') // '.'
102 [See release notes page on GitHub](https://github.com/es128/glob-parent/releases)
106 [ISC](https://raw.github.com/es128/glob-parent/master/LICENSE)
108 [expand-braces]: https://github.com/jonschlinkert/expand-braces
109 [expand-brackets]: https://github.com/jonschlinkert/expand-brackets