1 <img width="75px" height="75px" align="right" alt="Inquirer Logo" src="https://raw.githubusercontent.com/SBoudrias/Inquirer.js/master/assets/inquirer_readme.svg?sanitize=true" title="Inquirer.js"/>
5 [](http://badge.fury.io/js/inquirer)
6 [](http://travis-ci.org/SBoudrias/Inquirer.js)
7 [](https://codecov.io/gh/SBoudrias/Inquirer.js)
8 [](https://app.fossa.com/projects/git%2Bgithub.com%2FSBoudrias%2FInquirer.js?ref=badge_shield)
10 A collection of common interactive command line user interfaces.
14 1. [Documentation](#documentation)
15 1. [Installation](#installation)
16 2. [Examples](#examples)
17 3. [Methods](#methods)
18 4. [Objects](#objects)
19 5. [Questions](#questions)
20 6. [Answers](#answers)
21 7. [Separator](#separator)
22 8. [Prompt Types](#prompt)
23 2. [User Interfaces and Layouts](#layouts)
24 1. [Reactive Interface](#reactive)
25 3. [Support](#support)
26 4. [Known issues](#issues)
28 5. [Contributing](#contributing)
29 6. [License](#license)
30 7. [Plugins](#plugins)
32 ## Goal and Philosophy
34 **`Inquirer.js`** strives to be an easily embeddable and beautiful command line interface for [Node.js](https://nodejs.org/) (and perhaps the "CLI [Xanadu](https://en.wikipedia.org/wiki/Citizen_Kane)").
36 **`Inquirer.js`** should ease the process of
38 - providing _error feedback_
41 - _validating_ answers
42 - managing _hierarchical prompts_
44 > **Note:** **`Inquirer.js`** provides the user interface and the inquiry session flow. If you're searching for a full blown command line program utility, then check out [commander](https://github.com/visionmedia/commander.js), [vorpal](https://github.com/dthree/vorpal) or [args](https://github.com/leo/args).
46 ## [Documentation](#documentation)
48 <a name="documentation"></a>
52 <a name="installation"></a>
59 var inquirer = require('inquirer');
62 /* Pass your questions in here */
65 // Use user feedback for... whatever!!
68 if(error.isTtyError) {
69 // Prompt couldn't be rendered in the current environment
71 // Something else when wrong
76 <a name="examples"></a>
78 ### Examples (Run it and see it)
80 Check out the [`packages/inquirer/examples/`](https://github.com/SBoudrias/Inquirer.js/tree/master/packages/inquirer/examples) folder for code and interface examples.
83 node packages/inquirer/examples/pizza.js
84 node packages/inquirer/examples/checkbox.js
90 <a name="methods"></a>
92 #### `inquirer.prompt(questions) -> promise`
94 Launch the prompt interface (inquiry session)
96 - **questions** (Array) containing [Question Object](#question) (using the [reactive interface](#reactive-interface), you can also pass a `Rx.Observable` instance)
97 - returns a **Promise**
99 #### `inquirer.registerPrompt(name, prompt)`
101 Register prompt plugins under `name`.
103 - **name** (string) name of the this new prompt. (used for question `type`)
104 - **prompt** (object) the prompt object itself (the plugin)
106 #### `inquirer.createPromptModule() -> prompt function`
108 Create a self contained inquirer module. If you don't want to affect other libraries that also rely on inquirer when you overwrite or add new prompt types.
111 var prompt = inquirer.createPromptModule();
113 prompt(questions).then(/* ... */);
118 <a name="objects"></a>
122 <a name="questions"></a>
123 A question object is a `hash` containing question related values:
125 - **type**: (String) Type of the prompt. Defaults: `input` - Possible values: `input`, `number`, `confirm`,
126 `list`, `rawlist`, `expand`, `checkbox`, `password`, `editor`
127 - **name**: (String) The name to use when storing the answer in the answers hash. If the name contains periods, it will define a path in the answers hash.
128 - **message**: (String|Function) The question to print. If defined as a function, the first parameter will be the current inquirer session answers. Defaults to the value of `name` (followed by a colon).
129 - **default**: (String|Number|Boolean|Array|Function) Default value(s) to use if nothing is entered, or a function that returns the default value(s). If defined as a function, the first parameter will be the current inquirer session answers.
130 - **choices**: (Array|Function) Choices array or a function returning a choices array. If defined as a function, the first parameter will be the current inquirer session answers.
131 Array values can be simple `numbers`, `strings`, or `objects` containing a `name` (to display in list), a `value` (to save in the answers hash), and a `short` (to display after selection) properties. The choices array can also contain [a `Separator`](#separator).
132 - **validate**: (Function) Receive the user input and answers hash. Should return `true` if the value is valid, and an error message (`String`) otherwise. If `false` is returned, a default error message is provided.
133 - **filter**: (Function) Receive the user input and answers hash. Returns the filtered value to be used inside the program. The value returned will be added to the _Answers_ hash.
134 - **transformer**: (Function) Receive the user input, answers hash and option flags, and return a transformed value to display to the user. The transformation only impacts what is shown while editing. It does not modify the answers hash.
135 - **when**: (Function, Boolean) Receive the current user answers hash and should return `true` or `false` depending on whether or not this question should be asked. The value can also be a simple boolean.
136 - **pageSize**: (Number) Change the number of lines that will be rendered when using `list`, `rawList`, `expand` or `checkbox`.
137 - **prefix**: (String) Change the default _prefix_ message.
138 - **suffix**: (String) Change the default _suffix_ message.
139 - **askAnswered**: (Boolean) Force to prompt the question if the answer already exists.
140 - **loop**: (Boolean) Enable list looping. Defaults: `true`
142 `default`, `choices`(if defined as functions), `validate`, `filter` and `when` functions can be called asynchronously. Either return a promise or use `this.async()` to get a callback you'll call with the final value.
146 /* Preferred way: with promise */
148 return new Promise(/* etc... */);
151 /* Legacy way: with this.async */
152 validate: function (input) {
153 // Declare function as asynchronous, and save the done callback
154 var done = this.async();
157 setTimeout(function() {
158 if (typeof input !== 'number') {
159 // Pass the return value in the done callback
160 done('You need to provide a number');
163 // Pass the return value in the done callback
172 <a name="answers"></a>
173 A key/value hash containing the client answers in each prompt.
175 - **Key** The `name` property of the _question_ object
176 - **Value** (Depends on the prompt)
177 - `confirm`: (Boolean)
178 - `input` : User input (filtered if `filter` is defined) (String)
179 - `number`: User input (filtered if `filter` is defined) (Number)
180 - `rawlist`, `list` : Selected choice value (or name if no value specified) (String)
184 <a name="separator"></a>
185 A separator can be added to any `choices` array:
188 // In the question object
189 choices: [ "Choice A", new inquirer.Separator(), "choice B" ]
191 // Which'll be displayed this way
192 [?] What do you want to do?
197 Talk to the receptionist
200 The constructor takes a facultative `String` value that'll be use as the separator. If omitted, the separator will be `--------`.
202 Separator instances have a property `type` equal to `separator`. This should allow tools façading Inquirer interface from detecting separator types in lists.
204 <a name="prompt"></a>
210 > **Note:**: _allowed options written inside square brackets (`[]`) are optional. Others are required._
212 #### List - `{type: 'list'}`
214 Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties. (Note that
215 default must be the choice `index` in the array or a choice `value`)
217 
221 #### Raw List - `{type: 'rawlist'}`
223 Take `type`, `name`, `message`, `choices`[, `default`, `filter`, `loop`] properties. (Note that
224 default must be the choice `index` in the array)
226 
230 #### Expand - `{type: 'expand'}`
232 Take `type`, `name`, `message`, `choices`[, `default`] properties. (Note that
233 default must be the choice `index` in the array. If `default` key not provided, then `help` will be used as default choice)
235 Note that the `choices` object will take an extra parameter called `key` for the `expand` prompt. This parameter must be a single (lowercased) character. The `h` option is added by the prompt and shouldn't be defined by the user.
237 See `examples/expand.js` for a running example.
239 
240 
244 #### Checkbox - `{type: 'checkbox'}`
246 Take `type`, `name`, `message`, `choices`[, `filter`, `validate`, `default`, `loop`] properties. `default` is expected to be an Array of the checked choices value.
248 Choices marked as `{checked: true}` will be checked by default.
250 Choices whose property `disabled` is truthy will be unselectable. If `disabled` is a string, then the string will be outputted next to the disabled choice, otherwise it'll default to `"Disabled"`. The `disabled` property can also be a synchronous function receiving the current answers as argument and returning a boolean or a string.
252 
256 #### Confirm - `{type: 'confirm'}`
258 Take `type`, `name`, `message`, [`default`] properties. `default` is expected to be a boolean if used.
260 
264 #### Input - `{type: 'input'}`
266 Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
268 
272 #### Input - `{type: 'number'}`
274 Take `type`, `name`, `message`[, `default`, `filter`, `validate`, `transformer`] properties.
278 #### Password - `{type: 'password'}`
280 Take `type`, `name`, `message`, `mask`,[, `default`, `filter`, `validate`] properties.
282 
286 Note that `mask` is required to hide the actual user input.
288 #### Editor - `{type: 'editor'}`
290 Take `type`, `name`, `message`[, `default`, `filter`, `validate`] properties
292 Launches an instance of the users preferred editor on a temporary file. Once the user exits their editor, the contents of the temporary file are read in as the result. The editor to use is determined by reading the $VISUAL or $EDITOR environment variables. If neither of those are present, notepad (on Windows) or vim (Linux or Mac) is used.
294 <a name="layouts"></a>
296 ### Use in Non-Interactive Environments
297 `prompt()` requires that it is run in an interactive environment. (I.e. [One where `process.stdin.isTTY` is `true`](https://nodejs.org/docs/latest-v12.x/api/process.html#process_a_note_on_process_i_o)). If `prompt()` is invoked outside of such an environment, then `prompt()` will return a rejected promise with an error. For convenience, the error will have a `isTtyError` property to programmatically indicate the cause.
300 ## User Interfaces and layouts
302 Along with the prompts, Inquirer offers some basic text UI.
304 #### Bottom Bar - `inquirer.ui.BottomBar`
306 This UI present a fixed text at the bottom of a free text zone. This is useful to keep a message to the bottom of the screen while outputting command outputs on the higher section.
309 var ui = new inquirer.ui.BottomBar();
311 // pipe a Stream to the log zone
312 outputStream.pipe(ui.log);
314 // Or simply write output
315 ui.log.write('something just happened.');
316 ui.log.write('Almost over, standby!');
318 // During processing, update the bottom bar content to display a loader
319 // or output a progress bar, etc
320 ui.updateBottomBar('new bottom bar content');
323 <a name="reactive"></a>
325 ## Reactive interface
327 Internally, Inquirer uses the [JS reactive extension](https://github.com/ReactiveX/rxjs) to handle events and async flows.
329 This mean you can take advantage of this feature to provide more advanced flows. For example, you can dynamically add questions to be asked:
332 var prompts = new Rx.Subject();
333 inquirer.prompt(prompts);
335 // At some point in the future, push new questions
347 And using the return value `process` property, you can access more fine grained callbacks:
350 inquirer.prompt(prompts).ui.process.subscribe(onEachAnswer, onError, onComplete);
353 ## Support (OS Terminals)
355 <a name="support"></a>
357 You should expect mostly good support for the CLI below. This does not mean we won't
358 look at issues found on other command line - feel free to report any!
363 - **Windows ([Known issues](#issues))**:
364 - [ConEmu](https://conemu.github.io/)
368 - **Linux (Ubuntu, openSUSE, Arch Linux, etc)**:
369 - gnome-terminal (Terminal GNOME)
374 <a name="issues"></a>
376 Running Inquirer together with network streams in Windows platform inside some terminals can result in process hang.
377 Workaround: run inside another terminal.
378 Please refer to the https://github.com/nodejs/node/issues/21771
380 ## News on the march (Release notes)
384 Please refer to the [GitHub releases section for the changelog](https://github.com/SBoudrias/Inquirer.js/releases)
388 <a name="contributing"></a>
391 Unit test are written in [Mocha](https://mochajs.org/). Please add a unit test for every new feature or bug fix. `npm test` to run the test suite.
394 Add documentation for every API change. Feel free to send typo fixes and better docs!
396 We're looking to offer good support for multiple prompts and environments. If you want to
397 help, we'd like to keep a list of testers for each terminal/OS so we can contact you and
398 get feedback before release. Let us know if you want to be added to the list (just tweet
399 to [@vaxilart](https://twitter.com/Vaxilart)) or just add your name to [the wiki](https://github.com/SBoudrias/Inquirer.js/wiki/Testers)
403 <a name="license"></a>
405 Copyright (c) 2016 Simon Boudrias (twitter: [@vaxilart](https://twitter.com/Vaxilart))
406 Licensed under the MIT license.
410 <a name="plugins"></a>
414 [**autocomplete**](https://github.com/mokkabonna/inquirer-autocomplete-prompt)<br>
415 Presents a list of options as the user types, compatible with other packages such as fuzzy (for search)<br>
417 
419 [**checkbox-plus**](https://github.com/faressoft/inquirer-checkbox-plus-prompt)<br>
420 Checkbox list with autocomplete and other additions<br>
422 
424 [**datetime**](https://github.com/DerekTBrown/inquirer-datepicker-prompt)<br>
425 Customizable date/time selector using both number pad and arrow keys<br>
427 
429 [**inquirer-select-line**](https://github.com/adam-golab/inquirer-select-line)<br>
430 Prompt for selecting index in array where add new element<br>
432 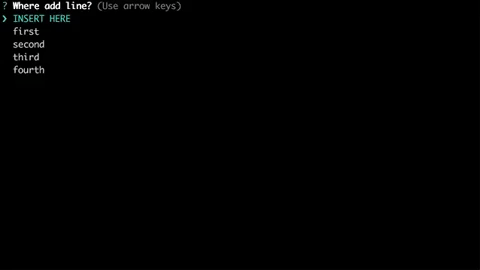
434 [**command**](https://github.com/sullof/inquirer-command-prompt)<br>
435 Simple prompt with command history and dynamic autocomplete<br>
437 [**inquirer-fuzzy-path**](https://github.com/adelsz/inquirer-fuzzy-path)<br>
438 Prompt for fuzzy file/directory selection.<br>
440 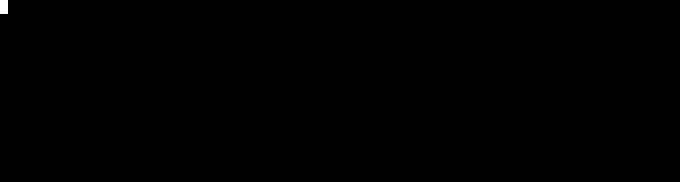
442 [**inquirer-emoji**](https://github.com/tannerntannern/inquirer-emoji)<br>
443 Prompt for inputting emojis.<br>
445 
447 [**inquirer-chalk-pipe**](https://github.com/LitoMore/inquirer-chalk-pipe)<br>
448 Prompt for input chalk-pipe style strings<br>
450 
452 [**inquirer-search-checkbox**](https://github.com/clinyong/inquirer-search-checkbox)<br>
453 Searchable Inquirer checkbox<br>
455 [**inquirer-search-list**](https://github.com/robin-rpr/inquirer-search-list)<br>
456 Searchable Inquirer list<br>
458 
460 [**inquirer-prompt-suggest**](https://github.com/olistic/inquirer-prompt-suggest)<br>
461 Inquirer prompt for your less creative users.<br>
463 
465 [**inquirer-s3**](https://github.com/HQarroum/inquirer-s3)<br>
466 An S3 object selector for Inquirer.<br>
468 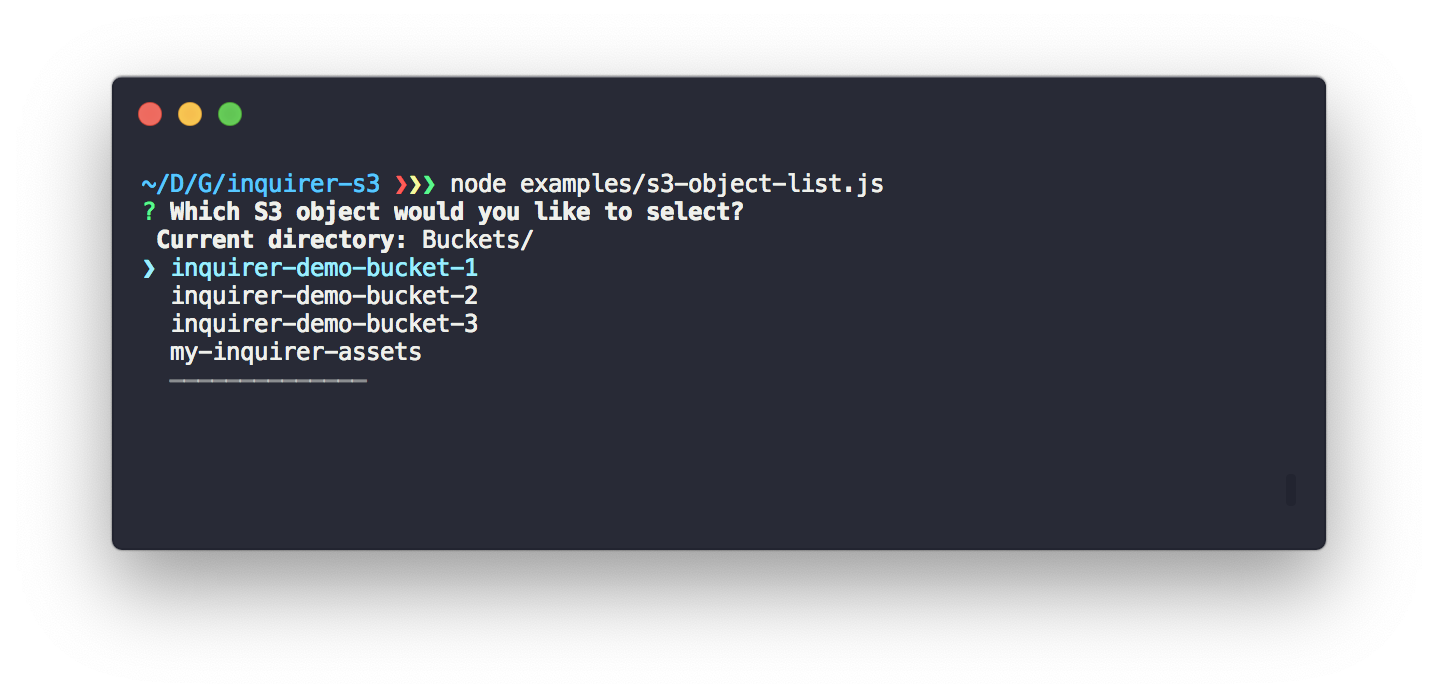
470 [**inquirer-autosubmit-prompt**](https://github.com/yaodingyd/inquirer-autosubmit-prompt)<br>
471 Auto submit based on your current input, saving one extra enter<br>
473 [**inquirer-file-tree-selection-prompt**](https://github.com/anc95/inquirer-file-tree-selection)<br>
474 Inquirer prompt for to select a file or directory in file tree<br>
476 
478 [**inquirer-table-prompt**](https://github.com/eduardoboucas/inquirer-table-prompt)<br>
479 A table-like prompt for Inquirer.<br>
481 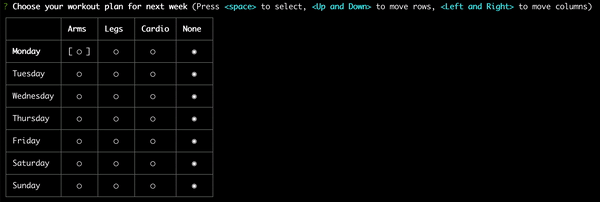