4 [](https://travis-ci.org/gajus/table)
5 [](https://coveralls.io/github/gajus/table)
6 [](https://www.npmjs.org/package/table)
7 [](https://github.com/gajus/canonical)
8 [](https://twitter.com/kuizinas)
11 * [Features](#table-features)
12 * [Install](#table-install)
13 * [Usage](#table-usage)
14 * [Cell Content Alignment](#table-usage-cell-content-alignment)
15 * [Column Width](#table-usage-column-width)
16 * [Custom Border](#table-usage-custom-border)
17 * [Draw Horizontal Line](#table-usage-draw-horizontal-line)
18 * [Single Line Mode](#table-usage-single-line-mode)
19 * [Padding Cell Content](#table-usage-padding-cell-content)
20 * [Predefined Border Templates](#table-usage-predefined-border-templates)
21 * [Streaming](#table-usage-streaming)
22 * [Text Truncation](#table-usage-text-truncation)
23 * [Text Wrapping](#table-usage-text-wrapping)
26 Produces a string that represents array data in a text table.
28 
30 <a name="table-features"></a>
33 * Works with strings containing [fullwidth](https://en.wikipedia.org/wiki/Halfwidth_and_fullwidth_forms) characters.
34 * Works with strings containing [ANSI escape codes](https://en.wikipedia.org/wiki/ANSI_escape_code).
35 * Configurable border characters.
36 * Configurable content alignment per column.
37 * Configurable content padding per column.
38 * Configurable column width.
41 <a name="table-install"></a>
49 [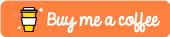](https://www.buymeacoffee.com/gajus)
50 [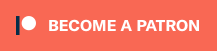](https://www.patreon.com/gajus)
52 <a name="table-usage"></a>
55 Table data is described using an array (rows) of array (cells).
63 // const {table} = require('table');
75 * @typedef {string} table~cell
79 * @typedef {table~cell[]} table~row
83 * @typedef {Object} table~columns
84 * @property {string} alignment Cell content alignment (enum: left, center, right) (default: left).
85 * @property {number} width Column width (default: auto).
86 * @property {number} truncate Number of characters are which the content will be truncated (default: Infinity).
87 * @property {number} paddingLeft Cell content padding width left (default: 1).
88 * @property {number} paddingRight Cell content padding width right (default: 1).
92 * @typedef {Object} table~border
93 * @property {string} topBody
94 * @property {string} topJoin
95 * @property {string} topLeft
96 * @property {string} topRight
97 * @property {string} bottomBody
98 * @property {string} bottomJoin
99 * @property {string} bottomLeft
100 * @property {string} bottomRight
101 * @property {string} bodyLeft
102 * @property {string} bodyRight
103 * @property {string} bodyJoin
104 * @property {string} joinBody
105 * @property {string} joinLeft
106 * @property {string} joinRight
107 * @property {string} joinJoin
111 * Used to dynamically tell table whether to draw a line separating rows or not.
112 * The default behavior is to always return true.
114 * @typedef {function} drawHorizontalLine
115 * @param {number} index
116 * @param {number} size
121 * @typedef {Object} table~config
122 * @property {table~border} border
123 * @property {table~columns[]} columns Column specific configuration.
124 * @property {table~columns} columnDefault Default values for all columns. Column specific settings overwrite the default values.
125 * @property {table~drawHorizontalLine} drawHorizontalLine
129 * Generates a text table.
131 * @param {table~row[]} rows
132 * @param {table~config} config
135 output = table(data);
152 <a name="table-usage-cell-content-alignment"></a>
153 ### Cell Content Alignment
155 `{string} config.columns[{number}].alignment` property controls content horizontal alignment within a cell.
157 Valid values are: "left", "right" and "center".
187 output = table(data, config);
193 ╔════════════╤════════════╤════════════╗
195 ╟────────────┼────────────┼────────────╢
197 ╟────────────┼────────────┼────────────╢
199 ╚════════════╧════════════╧════════════╝
202 <a name="table-usage-column-width"></a>
205 `{number} config.columns[{number}].width` property restricts column width to a fixed width.
226 output = table(data, options);
232 ╔════╤════════════╤════╗
234 ╟────┼────────────┼────╢
236 ╟────┼────────────┼────╢
238 ╚════╧════════════╧════╝
241 <a name="table-usage-custom-border"></a>
244 `{object} config.border` property describes characters used to draw the table border.
280 output = table(data, config);
295 <a name="table-usage-draw-horizontal-line"></a>
296 ### Draw Horizontal Line
298 `{function} config.drawHorizontalLine` property is a function that is called for every non-content row in the table. The result of the function `{boolean}` determines whether a row is drawn.
315 * @typedef {function} drawHorizontalLine
316 * @param {number} index
317 * @param {number} size
320 drawHorizontalLine: (index, size) => {
321 return index === 0 || index === 1 || index === size - 1 || index === size;
325 output = table(data, options);
344 <a name="table-usage-single-line-mode"></a>
347 Horizontal lines inside the table are not drawn.
356 ['-rw-r--r--', '1', 'pandorym', 'staff', '1529', 'May 23 11:25', 'LICENSE'],
357 ['-rw-r--r--', '1', 'pandorym', 'staff', '16327', 'May 23 11:58', 'README.md'],
358 ['drwxr-xr-x', '76', 'pandorym', 'staff', '2432', 'May 23 12:02', 'dist'],
359 ['drwxr-xr-x', '634', 'pandorym', 'staff', '20288', 'May 23 11:54', 'node_modules'],
360 ['-rw-r--r--', '1,', 'pandorym', 'staff', '525688', 'May 23 11:52', 'package-lock.json'],
361 ['-rw-r--r--@', '1', 'pandorym', 'staff', '2440', 'May 23 11:25', 'package.json'],
362 ['drwxr-xr-x', '27', 'pandorym', 'staff', '864', 'May 23 11:25', 'src'],
363 ['drwxr-xr-x', '20', 'pandorym', 'staff', '640', 'May 23 11:25', 'test'],
370 const output = table(data, config);
375 ╔═════════════╤═════╤══════════╤═══════╤════════╤══════════════╤═══════════════════╗
376 ║ -rw-r--r-- │ 1 │ pandorym │ staff │ 1529 │ May 23 11:25 │ LICENSE ║
377 ║ -rw-r--r-- │ 1 │ pandorym │ staff │ 16327 │ May 23 11:58 │ README.md ║
378 ║ drwxr-xr-x │ 76 │ pandorym │ staff │ 2432 │ May 23 12:02 │ dist ║
379 ║ drwxr-xr-x │ 634 │ pandorym │ staff │ 20288 │ May 23 11:54 │ node_modules ║
380 ║ -rw-r--r-- │ 1, │ pandorym │ staff │ 525688 │ May 23 11:52 │ package-lock.json ║
381 ║ -rw-r--r--@ │ 1 │ pandorym │ staff │ 2440 │ May 23 11:25 │ package.json ║
382 ║ drwxr-xr-x │ 27 │ pandorym │ staff │ 864 │ May 23 11:25 │ src ║
383 ║ drwxr-xr-x │ 20 │ pandorym │ staff │ 640 │ May 23 11:25 │ test ║
384 ╚═════════════╧═════╧══════════╧═══════╧════════╧══════════════╧═══════════════════╝
387 <a name="table-usage-padding-cell-content"></a>
388 ### Padding Cell Content
390 `{number} config.columns[{number}].paddingLeft` and `{number} config.columns[{number}].paddingRight` properties control content padding within a cell. Property value represents a number of whitespaces used to pad the content.
398 ['0A', 'AABBCC', '0C'],
415 output = table(data, config);
432 <a name="table-usage-predefined-border-templates"></a>
433 ### Predefined Border Templates
435 You can load one of the predefined border templates using `getBorderCharacters` function.
453 border: getBorderCharacters(`name of the template`)
480 # ramac (ASCII; for use in terminals that do not support Unicode characters)
490 # void (no borders; see "bordless table" section of the documentation)
500 Raise [an issue](https://github.com/gajus/table/issues) if you'd like to contribute a new border template.
502 <a name="table-usage-predefined-border-templates-borderless-table"></a>
503 #### Borderless Table
505 Simply using "void" border character template creates a table with a lot of unnecessary spacing.
507 To create a more plesant to the eye table, reset the padding and remove the joining rows, e.g.
512 output = table(data, {
513 border: getBorderCharacters(`void`),
518 drawHorizontalLine: () => {
532 <a name="table-usage-streaming"></a>
535 `table` package exports `createStream` function used to draw a table and append rows.
537 `createStream` requires `{number} columnDefault.width` and `{number} columnCount` configuration properties.
554 stream = createStream(config);
557 stream.write([new Date()]);
561 
563 `table` package uses ANSI escape codes to overwrite the output of the last line when a new row is printed.
565 The underlying implementation is explained in this [Stack Overflow answer](http://stackoverflow.com/a/32938658/368691).
567 Streaming supports all of the configuration properties and functionality of a static table (such as auto text wrapping, alignment and padding), e.g.
574 import _ from 'lodash';
599 stream = createStream(config);
606 random = _.sample('abcdefghijklmnopqrstuvwxyz', _.random(1, 30)).join('');
608 stream.write([i++, new Date(), random]);
612 
614 <a name="table-usage-text-truncation"></a>
617 To handle a content that overflows the container width, `table` package implements [text wrapping](#table-usage-text-wrapping). However, sometimes you may want to truncate content that is too long to be displayed in the table.
619 `{number} config.columns[{number}].truncate` property (default: `Infinity`) truncates the text at the specified length.
627 ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
639 output = table(data, config);
645 ╔══════════════════════╗
646 ║ Lorem ipsum dolor si ║
647 ║ t amet, consectetur ║
648 ║ adipiscing elit. Pha ║
649 ║ sellus pulvinar nibh ║
650 ║ sed mauris conva... ║
651 ╚══════════════════════╝
654 <a name="table-usage-text-wrapping"></a>
657 `table` package implements auto text wrapping, i.e. text that has width greater than the container width will be separated into multiple lines, e.g.
665 ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
676 output = table(data, config);
682 ╔══════════════════════╗
683 ║ Lorem ipsum dolor si ║
684 ║ t amet, consectetur ║
685 ║ adipiscing elit. Pha ║
686 ║ sellus pulvinar nibh ║
687 ║ sed mauris convallis ║
688 ║ dapibus. Nunc venena ║
689 ║ tis tempus nulla sit ║
691 ╚══════════════════════╝
694 When `wrapWord` is `true` the text is broken at the nearest space or one of the special characters ("-", "_", "\", "/", ".", ",", ";"), e.g.
702 ['Lorem ipsum dolor sit amet, consectetur adipiscing elit. Phasellus pulvinar nibh sed mauris convallis dapibus. Nunc venenatis tempus nulla sit amet viverra.']
714 output = table(data, config);
720 ╔══════════════════════╗
721 ║ Lorem ipsum dolor ║
725 ║ Phasellus pulvinar ║
727 ║ convallis dapibus. ║
731 ╚══════════════════════╝