3 Snippets solution for [coc.nvim](https://github.com/neoclide/coc.nvim)
5 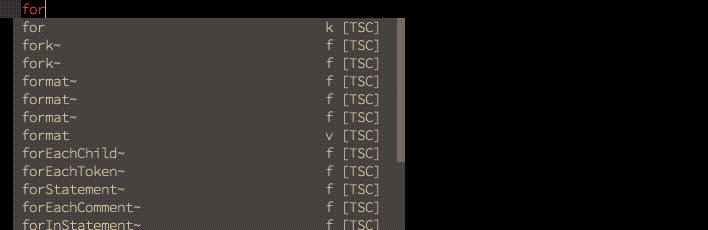
7 _Snippet preview requires [neovim 0.4 or latest vim8](https://github.com/neoclide/coc.nvim/wiki/F.A.Q#how-to-make-preview-window-shown-aside-with-pum)_
11 - Load UltiSnips snippets.
12 - Load snipmate snippets.
13 - Load VSCode snippets from coc extensions.
14 - Load VSCode snippets from custom directories.
15 - Load UltiSnips snippets from configured folder.
16 - Provide snippets as completion items.
17 - Provide expand and expandOrJump keymaps for snippet.
18 - Provide snippets list for edit snippet.
19 - Provide `snippets.editSnippets` command for edit user snippets of current filetype.
21 **Note:** some features of ultisnips and snipmate format snippets not supported, checkout [faq](#faq).
25 - Use same keys for jump placeholder.
26 - Nested snippet support.
27 - Always async, never slows you down.
28 - Improved match for complete items with TextEdit support.
29 - Edit snippets of current buffer by `:CocList snippets`, sorted by mru.
33 If you like my work, consider supporting me on Patreon or PayPal:
35 <a href="https://www.patreon.com/chemzqm"><img src="https://c5.patreon.com/external/logo/become_a_patron_button.png" alt="Patreon donate button" /> </a>
36 <a href="https://www.paypal.com/paypalme/chezqm"><img src="https://werwolv.net/assets/paypal_banner.png" alt="PayPal donate button" /> </a>
40 In your vim/neovim, run command:
43 :CocInstall coc-snippets
49 " Use <C-l> for trigger snippet expand.
50 imap <C-l> <Plug>(coc-snippets-expand)
52 " Use <C-j> for select text for visual placeholder of snippet.
53 vmap <C-j> <Plug>(coc-snippets-select)
55 " Use <C-j> for jump to next placeholder, it's default of coc.nvim
56 let g:coc_snippet_next = '<c-j>'
58 " Use <C-k> for jump to previous placeholder, it's default of coc.nvim
59 let g:coc_snippet_prev = '<c-k>'
61 " Use <C-j> for both expand and jump (make expand higher priority.)
62 imap <C-j> <Plug>(coc-snippets-expand-jump)
64 " Use <leader>x for convert visual selected code to snippet
65 xmap <leader>x <Plug>(coc-convert-snippet)
68 Make `<tab>` used for trigger completion, completion confirm, snippet expand and jump like VSCode.
71 inoremap <silent><expr> <TAB>
72 \ pumvisible() ? coc#_select_confirm() :
73 \ coc#expandableOrJumpable() ? "\<C-r>=coc#rpc#request('doKeymap', ['snippets-expand-jump',''])\<CR>" :
74 \ <SID>check_back_space() ? "\<TAB>" :
77 function! s:check_back_space() abort
78 let col = col('.') - 1
79 return !col || getline('.')[col - 1] =~# '\s'
82 let g:coc_snippet_next = '<tab>'
85 **Note:** `coc#_select_confirm()` helps select first complete item when there's
86 no complete item selected, neovim 0.4 or latest vim8 required for this function
91 Some ultisnips features are **not** supported:
93 - [x] Position check of trigger option, including `b`, `w` and `i`.
94 - [x] Execute vim, python and shell code in snippet.
95 - [x] `extends`, `priority` and `clearsnippets` command in snippet file.
96 - [x] Visual placeholder.
97 - [x] Placeholder and variable transform.
98 - [x] Expression snippet.
99 - [x] Automatic trigger snippet.
100 - [x] Context snippets.
101 - [x] Support loading snipmate snippets.
102 - [ ] Execute shell code with custom shabang (will not support).
103 - [ ] Automatic reformat snippet after change of placeholder (can't support).
104 - [ ] Format related snippet options, including `t`, `s` and `m` (can't support).
105 - [ ] Snippet actions (can't support).
107 **Note**: python regex in snippet are converted to javascript regex, however,
108 some regex patterns can't be supported by javascript, including
109 `\u` `(?s)` `\Z` `(?(id/name)yes-pattern|no-pattern)`.
113 - `snippets.priority`: priority of snippets source, default `90`.
114 - `snippets.editSnippetsCommand`: Open command used for snippets.editSnippets command, use coc.preferences.jumpCommand by default.
115 - `snippets.trace`: Trace level of snippets channel.
116 - `snippets.enableStatusItem`: Enable status item in `g:coc_status` used for statusline.
117 - `snippets.extends`: extends filetype's snippets with other filetypes, example:
122 "javascriptreact": ["javascript"],
123 "typescript": ["javascript"]
127 - `snippets.userSnippetsDirectory`, Directory that contains custom user ultisnips snippets, use ultisnips in extension root by default.
128 - `snippets.shortcut`, shortcut in completion menu, default `S`.
129 - `snippets.autoTrigger`: enable auto trigger for auto trigger ultisnips snippets, default `true`.
130 - `snippets.triggerCharacters`: trigger characters for completion, default `[]`.
131 - `snippets.loadFromExtensions`: load snippets from coc.nvim extensions, default: `true`.
132 - `snippets.textmateSnippetsRoots`: absolute directories that contains textmate/VSCode snippets to load.
133 - `snippets.ultisnips.enable`: enable load UltiSnips snippets, default `true`.
134 - `snippets.ultisnips.usePythonx`: use `pythonx` for eval python code when possible, default `true`.
135 - `snippets.ultisnips.pythonVersion`: when `usePythonx` is false, python version to use for
136 python code, default to `3`.
137 - `snippets.ultisnips.directories`: directories that searched for snippet files,
138 could be subfolder in every \$runtimepath or absolute paths, default: `["UltiSnips"]`
139 - `snippets.snipmate.enable`: enable load snipmate snippets, default `true`.
140 - `snippets.snipmate.author`: author name used for `g:snips_author`
144 - Use `:CocList snippets` to open snippets list.
145 - Use `:CocCommand snippets.editSnippets` to edit user snippet of current filetype.
146 - Use `:CocCommand snippets.openSnippetFiles` to open snippet files of current filetype.
150 **Q:** How to check if a snippet successfully loaded?
152 **A:** Use command `:CocCommand workspace.showOutput snippets`
154 **Q:** Some ultisnips snippet not works as expected.
156 **A:** Reformat after change of placeholder feature can't be supported for now,
157 and some regex pattern can't be converted to javascript regex pattern, so the
158 snippet can be failed to load.
160 **Q:** Where to get snippets?
162 **A:** One solution is install [honza/vim-snippets](https://github.com/honza/vim-snippets) which is widely used.
164 **Q:** Do I need to install [Ultisnips](https://github.com/SirVer/ultisnips).
166 **A:** No! This extension is designed to work with or without Ultisnips, you can
167 still install Ultisnips, but this extension would not run any code or read
168 configuration from it.
170 **Q:** How to check jumpable or expandable at current position.
172 **A:** Use functions provided by coc.nvim: `coc#expandable()` `coc#jumpable()` and `coc#expandableOrJumpable()`.
174 **Q:** It doesn't load snippets from [vim-go](https://github.com/fatih/vim-go).
176 **A:** It uses `g:UltiSnipsSnippetDirectories` which is not supported, you can
180 snippets.ultisnips.directories: [
182 "gosnippets/UltiSnips"
188 **Q:** How could I add custom UltiSnips snippets.
190 **A:** You can create snippet files in folder: `$VIMCONFIG/coc/ultisnips`, use
191 command `:CocCommand snippets.editSnippets` to open user snippet of current